Hi Friends, in past we were using third party library for Paypal payment but now Paypal provide us their own library for payment so it is now much secure and easy to implement in our application. Below are the important step to do -
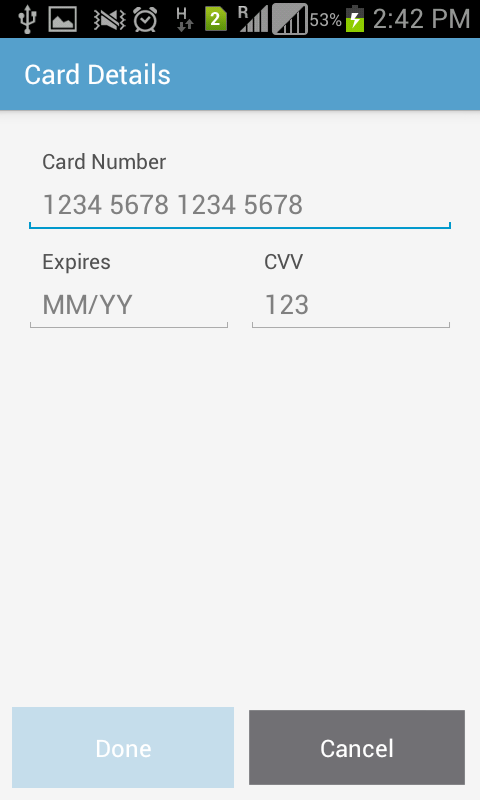
1)First go through Paypal Developer web site and create an application.
2)Now open your manifest file and give the below permissions-
<uses-permission android:name="android.permission.INTERNET"
/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"
/>
3)And some required Activity and Services-
<service
android:name="com.paypal.android.sdk.payments.PayPalService"
android:exported="false" />
<activity android:name="com.paypal.android.sdk.payments.PaymentActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.LoginActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.PaymentMethodActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.PaymentConfirmActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.PayPalFuturePaymentActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.FuturePaymentConsentActivity"
/>
<activity android:name="com.paypal.android.sdk.payments.FuturePaymentInfoActivity"
/>
<activity
android:name="io.card.payment.CardIOActivity"
android:configChanges="keyboardHidden|orientation" />
<activity android:name="io.card.payment.DataEntryActivity"
/>
//set the environment for
production/sandbox/no netowrk
private static final String CONFIG_ENVIRONMENT =
PayPalConfiguration.ENVIRONMENT_PRODUCTION;
6)Inside onCreate method call the Paypal service-
Intent intent = new Intent(this, PayPalService.class);
intent.putExtra(PayPalService.EXTRA_PAYPAL_CONFIGURATION, config);
startService(intent);
7)Now you are ready to make a payment just on button press call the Payment Activity-
PayPalPayment thingToBuy = new PayPalPayment(new BigDecimal(1),"USD", "androidhub4you.com",
PayPalPayment.PAYMENT_INTENT_SALE);
Intent intent = new Intent(MainActivity.this, PaymentActivity.class);
intent.putExtra(PaymentActivity.EXTRA_PAYMENT, thingToBuy);
startActivityForResult(intent, REQUEST_PAYPAL_PAYMENT);
8)And finally from the onActivityResult get the payment response-
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent
data) {
if (requestCode == REQUEST_PAYPAL_PAYMENT) {
if (resultCode == Activity.RESULT_OK) {
PaymentConfirmation confirm =
data
.getParcelableExtra(PaymentActivity.EXTRA_RESULT_CONFIRMATION);
if (confirm != null) {
try {
System.out.println("Responseeee"+confirm);
Log.i("paymentExample",
confirm.toJSONObject().toString());
JSONObject jsonObj=new
JSONObject(confirm.toJSONObject().toString());
String
paymentId=jsonObj.getJSONObject("response").getString("id");
System.out.println("payment
id:-=="+paymentId);
Toast.makeText(getApplicationContext(),
paymentId, Toast.LENGTH_LONG).show();
} catch (JSONException e) {
Log.e("paymentExample", "an extremely
unlikely failure occurred: ", e);
}
}
} else if (resultCode ==
Activity.RESULT_CANCELED) {
Log.i("paymentExample", "The user
canceled.");
} else if (resultCode ==
PaymentActivity.RESULT_EXTRAS_INVALID) {
Log.i("paymentExample", "An invalid
Payment was submitted. Please see the docs.");
}
}
}
Very nice article. Thank you
ReplyDeleteYour welcome and thanks Ravi.
DeleteThis comment has been removed by the author.
Deletegood and hope u will post some more --------
DeleteYes sure i will post and thank you..
Deleteis it against google policy to accept money via pay pal?
ReplyDeleteNo.. is it mention any where? If it is against goole policy then why paypal have libray for android? As per my knowladge paypal is accepted by google.
DeleteNo.. is it mention any where? If it is against goole policy then why paypal have libray for android? As per my knowladge paypal is accepted by google.
Deletejust wanted to know coz goofle has monopoly they can ban u for no reason
Deletehello,
DeleteI want to implement the payment gateway in my SAPUI5 application..
Please suggest me the proper solution
Thanks
Vaidehi
Hi Manish ...Thanks for ur awesome tutorials on uncommon & nice topics... This tutorial was great too. Please let me know if you have any prior experience with paypal...Do they accept indian cards? And ...Only these steps you mentioned are required to start online transactions.
ReplyDeleteIndian cards means? well try this demo app it will accept all type of common card like Visa, Master etc.
DeleteCan you check my code here http://bit.ly/1uZ1r42
ReplyDeletei am getting invalid merchant clientId, Thanks
you are using the Classic API Username instead of the application client_id. To fix, log in to developer.paypal.com, navigate to Applications > My Apps > the name of your app. Open the 'REST API CREDENTIALS ' window, and you'll see a sandbox and live client_id. Copy the appropriate id into your app.
DeleteHi manish thanks for the above tutorial... and i have few queries... i have done all the above steps but my response remains same i.e pay ID and created_time... why so? and how will i know that the payment has happened? (will there be any mail sent or invoice to acknowledge my transfer) and i have already droped question in stack overflow regarding this(http://stackoverflow.com/questions/28043316/paypal-integration-android)... if you could help then it will be great thanks...
ReplyDeletesir this is no doubt very nice article but when i start on emultor then it shows ur device is not registered
ReplyDeleteI think this code will not work on emulator, try over the real phone.
Delete"config cannot be resolved to a variable" Here you can't created 'config' variable.
ReplyDeleteprivate static PayPalConfiguration config = new PayPalConfiguration()
Delete.environment(CONFIG_ENVIRONMENT)
.clientId(CONFIG_CLIENT_ID)
.acceptCreditCards(true)
.languageOrLocale("EN")
.rememberUser(true)
.merchantName("xyz");
Any Tutorial For Payumoney integration??please help me. or any other tutorial for pay in ruppe???? suggest tutorial.thank u
ReplyDeleteUnauthorized Device
ReplyDeletepayments from this device are not allowed.
How can I solve this problem .
are you using real device or emulator?
DeleteEmulator
DeleteUnauthorized Device
ReplyDeletepayments from this device are not allowed.
How can I solve this problem .
Tested in Real Device
how to implement Android studio
ReplyDeletefor me unfortunately its closing
ReplyDeleteCan you share crash report? And check your permissions in manifest file.
Deletehow can i integrate google wallet in android
ReplyDeleteYou can try below link-
Deletehttp://www.androidhub4you.com/2013/03/how-to-inegrate-in-app-purchase-billing.html
Note: It is 2013 code so if Google have been changed their way to doing thing, please visit Google Developer Blogs.
Hi Manish, I am getting "The merchant does not accept payments of this type." while paying through MasterCard credit card.
ReplyDeleteHow can i solve this problem.
Are you using sandbox mode or live? And please check the country if they accept the card payment through PayPal. For more detail visit PayPal developer blog.
DeleteThanks for reply..!
ReplyDeleteI am using production environment with client id of production and business account from Singapore.
hello sir please guide me that how can i start ios development on windows pc. i tried to find but still i fail in googling so please help me to any third party tool which can help me to start ios development on windows pc
ReplyDeleteLegally you can't do that.Any how if you able to use xcode and developing the application but still you will not be able to upload your application on apple store.
DeleteAny Tutorial For Payumoney integration??
ReplyDeleteplease help me.
Suggest me the proper solution or tutorial.
Thanks
Vaidehi
Nope, sorry.
DeleteHie manish sir can i get your client id for testing because with my client id it shows invalid client id please reply soon
ReplyDeleteI am really sorry but I can't share client id because of security reason. Please try to debug your setting and see why you are getting this error.
DeleteThis comment has been removed by the author.
ReplyDeletei tried your code work like a charm but my question i want to store that cut balance in other paypal account what should i do??
ReplyDeleteI think for security reason you can't. You need to make an app on Paypal account and only that account you can use for transaction.
DeleteHello manish plz help me android paypal create full source code send me plz help me sir this is my email_id m.manivelmca@gmail.com
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHello manish plz help me android paypal create full source code send me plz help me sir this is my email_id m.manivelmca@gmail.com
ReplyDeletePlease download the zip code from GIT-
Deletehttps://github.com/manishsri01/PayPalDemo
pay with card page is not opening.
ReplyDelete1)Please check log cat what error you are getting.
Delete2)Please check paypal documents in which country they support card payment.
hi...
ReplyDeletepayumoney android studio
hello sir..
ReplyDeletei want to implement parallel paypal payment in my android application.could you help me please.
This comment has been removed by the author.
ReplyDelete